How to Check Connectivity to a Target Network using Ping in Python
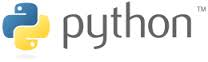
In this article, we show how to check connectivity to a target network using ping in Python.
Ping is a way of sending packets to a target network using Internet Control Message Protocols (ICMP) packets.
Ping operates by a simple principle of the source machine sending an ICMP echo request and the target machine replying with an ICMP echo reply. Hence, the implementation of ping is sometimes also called an Echo Server. This is also one of the first programs one writes while learning socket programming.
Ping uses ICMP to send ECHO request packets to the target system and expects a response within a certain time threshold.
It is used frequently to test network connectivity and troubleshoot network problems. For example, you can ping from various machines to test if incoming and outgoing packets are allowed. You can use ping from inside a virtual machine to test if an external website (like your bank's) is accessible, confirming if the internet can be accessed from the internal private network. If ping fails to reach a host, either there is no internet connection or the domain's server may be down.
In order to send a ping request in Python, you must download the pythonping module.
This is can through the following line of code.
pip install pythonping
So the program to ping a target network is shown below. In this case, the website we use is 'bing.com'.
So let's now go over the code.
First, we have to import the pythonping module before we can use it.
We create a class, PingConnectivity.
We then create a function, check_ping_connectivity(), which pings the host computer.
We then have our main function, which runs the code to ping to bing.com in this instance.
A much simpler version of this code is shown below.
The code above produces the following output shown below.
You can see that the code creates 4 separate pings to the server that hosts bing.com.
The IP address is the same for each one.
The reply packets are 29 bytes each.
The time to get the reply response for each is specified in ms (milliseconds).
So we can see from this code that we were able to establish connectivity with our target network.
Pinging a network is how we know if we can reach a network or not.
A failed response would produce an error message such as that shown below.
This specific error message above occurred because there was no internet connection but you would get the same error message if the target network could not be reached.
If you were to disconnect your internet or type in a domain name that doesn't exist, you will get this error message.
You can also modify the code by specifying various parameters, such as the number of pings that you want to send.
By default, 4 are sent, but if you want to send more such as to investigate the time it takes for each reply packet to be sent
back, you can use the count parameter and specify the number there.
We will now get 10 reply packets, which is shown below.
Other parameters that you can specify are include the size (which is the size of the ICMP payload you desire), and the timeout (which is the number of seconds you wish to wait for a response before assuming the target is unreachable).
To find all the parameters, you can look at the official page for pythonping, which can be found at the following link: https://pypi.org/project/pythonping/
Pinging this website, learningaboutelectronics.com, produces the following output.
And this is a common method of checking connectivity to a target system using ping in Python.
Related Resources
How to Randomly Select From or Shuffle a List in Python