How to Scan for Open Ports and Services using nmap in Python
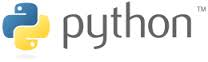
In this article, we show how to scan for open ports and services using the nmap module in Python.
NMAP, or Network Mapper, is a network scanner that can discover hosts and services on a computer network.
And with the help of various in-built scripts that can be executed using specific parameters, NMAP can also find out other information such as ciphers, specific vulnerabilites, etc.
More information about NMAP can be found at https://nmap.org
Documentation for NMAP can be found at https://nmap.org
Before we start on this Python program, the first thing you must do is install the nmap software.
This is not simply using pip, but the actual nmap software for your particular operating system.
For windows, the best way of doing this is going to nmap's website at https://nmap.org/download.html#windows and selecting the latest stable release of the .exe file. From there, you can install it onto your system.
Once this is in place and the nmap is in your PATH variable, you should be able to go to the
windows command prompt and type in 'nmap'. If you do so, you should see the version and other information
such as that shown below.
If this doesn't appear, then nmap must be placed in the PATH variable.
After this, then we must install the nmap module in Python,
which can be done with the line below.
pip install python3-nmap
Once this is done, we have everything we need to run nmap commands in Python.
So we go to our code.
So let's now go over the code.
First, we have to import nmap3 and json
We create a class, NmapPortScanner
We then create a function, port_scan(), which prints out the top ports of the nmap scan.
The scan_top_ports() function scans the top ports of the target system, which in this case is 'bing.com'.
We then have our main function, which executes the code.
A simpler version of this code is shown below.
The code above produces the following output shown below.
So we can see that the very top port is port 21, which is a TCP protocol. This is an open port, which means that an application on the target machine is listening for connections/packets on that port. This is a FTP port, which is a file transfer protocol. Files can be uploaded to the server through this port.
The second port is port 22, which is a TCP protocol. This is a filtered port which means that a firewall, filter, or other network obstacle is blocking the port so that Nmap cannot tell whether it is open or closed. This is a SSH port, which is secure shell, a software package that enables secure system administration and file transfers over insecure networks.
The third port is port 23, whcih is a TCP protocol. This also is a filtered port. This is a telnet port, which is a text-based network protocol that facilitates remote computer access.
The fourth port is port 25, which is a TCP protocol. This is a filtered port. This is a smtp port, which is Simple Mail Transfer Protocol used in sending and receiving email. SMTP is used most commonly by email clients, including Gmail, Outlook, Apple Mail and Yahoo Mail.
The fifth port is port 80, which is a TCP protocol. The port is open. This is a HTTP port, which is a protocol for fetching resources such as HTML documents. It is the foundation of any data exchange on the Web and it is a client-server protocol, which means requests are initiated by the recipient, usually the Web browser.
The sixth port is port 110, which is a TCP protocol. The port is filtered. This is a POP3 port, which is a protocol for receiving email by downloading it to your computer from a mailbox on the server of an internet service provider.
The seventh port is port 139, which is a TCP protocol. The port is filtered. This is a netbios-ssn port, which is a service facilitates connection-oriented communication between devices in a LAN, allowing the exchange of data through established sessions.
The eighth port is port 443, which is a TCP protocol. The port is open. This is a HTTPS port, which is a protocol just like HTTP but is encrypted for secure transmission.
The ninth port is port 445, which uses the TCP protocol. The port is filtered. This is a micrsoft-ds port, which is used for Server Message Blocks (SMB). The Server Message Block protocol (SMB protocol) is a client-server communication protocol used for sharing access to files, printers, serial ports and other resources on a network. It can also carry transaction protocols for interprocess communication.
The tenth port is port 3389, which uses the TCP protocol. Teh port is filtered. This is a ms-wbt-server port, which is used for used for Windows Remote Desktop and Remote Assistance connections (RDP- Remote Desktop Protocol) and also used by Windows Terminal Server.
This gives you a rundown for the top 10 ports used by a Microsoft website.
If you don't want to limit yourself to just the top 10 ports, then, with the scan_top_ports() function, you specify the number of ports that you want to scan by entering a number with the default parameter.
If, for instance, we wanted to scan the top 20 ports on the bing.com web server, we would
do so with the following line below.
This will now give you the top 20 ports instead of just the default top 10.
There are all type of command-line instructions that you can give, so that you filter out certain ports and only select certain ones.
This documentation can be viewed at https://nmap.org/book/port-scanning-options.html
If you want to find only the ports that are open on the server that hosts bing, you would use the following line of code shown below.
This would produce the following output shown below.
If you didn't specify a default of 1000, then it would only search the first 10 top ports for those whose state are open.
So we can see that there are 4 ports that have an open state out of the 1000 scanned: ftp, http, https, and h323q931.
All the other ports are either not open or mixed.
And this is how you can scan for open ports and services using nmap in Python.
Related Resources
How to Randomly Select From or Shuffle a List in Python